Mahir Koding – Queue adalah bentuk lain dari konsep implementasi linked list. Berbeda dengan Stack, yang menerapkan konsep LIFO (Last In First Out),Queue justru mempunyai konsep yang berbeda yakni FIFO (First In First Out). Setiap data yang pertama kali masuk, dialah yang akan keluar duluan. Contoh paling simple dalam kehidupan sehari hari adalah antrian pengunjung bank. Biasanya saat masuk, kita akan diberi nomor antrian terlebih dahulu sebelum dipanggil oleh teller. Kita akan menunggu sampai urutan kita barulah kita dapat bertransaksi di teller.
Linked list adalah suatu cara untuk menyimpan suatu data dengan struktur sehingga program dapat secara otomatis menciptakan suatu tempat baru untuk menyimpan suatu data kapan saja diperlukan. Secara rinci program dapat menuliskan struct atau definisi kelas yang berisi variabel yang memegang informasi yang ada didalamnya, dan mempunyai suatu. Contoh Program Queue Dengan Linked List In Word Contoh Program Queue Dengan Linked List Example Program kali ini cukup istimewa karena menggunakan class sebagai dasar dari OOP(Object Oriented Programing) berikut source code dari program queue menggunakan linked list. May 18, 2019 Contoh Program Queue Dengan Linked List Rating: 9,5/10 3107 reviews Dalam contoh program berikut ini saya gunakan double linked list untuk implementasi queue. Secara umum, operasi dalam queue ada 2 yang utama yaitu enqueue dan dequeue.
Dalam implementasi Queue ini, kita akan menggunakan Push Head dan Pop Tail.
http://techwelkin.com/wp-content/uploads/2016/04/fifo-queue-techwelkin.png
Berikut adalah contoh source codenya :
Metode linked list yang digunakan adalah double linked list. Operasi-operasi Queue dengan Double Linked List IsEmpty Fungsi IsEmpty berguna untuk mengecek apakah queue masih kosong atau sudah berisi data. Hal ini dilakukan dengan mengecek apakah head masih menunjukkan pada Null atau tidak. Today we are going to write a program to implement queue using linked list C, let’s start with what is queue: What is QUEUE? A Queue is a particular kind of abstract data type or collection in which the entities in the collection are kept in order and the principal (or only) operations on the collection are the addition of entities to the rear terminal position, known as enqueue, and removal.
Source code secara lengkap bisa dicek ke github saya, di link ini.
Jika ada pertanyaan yang kurang jelas silahkan berkomentar di bawah. Atau, jika ingin request tutorial juga dapat ke halaman ini. Dukung terus Mahir Koding agar dapat selalu mengupdate artikel dengan share dan like artikel ini. Terima Kasih.
Notice: Undefined offset: 0 in /var/www/mahirkoding/wp-content/themes/portus-premium-theme/includes/single/about-author.php on line 25
I have some problems that code. I read file in code and build one stack and one queues structure. But the code wasn't run correctly.
This is Node classI used Double LinkedList
** this is stack class. **
This is Queues Class
- Kali ini mimin ingin share Contoh Program single linked list dengan C++. Sintax kali ini masih sangat sederhana sebab ini request yang san.
- Program Implementasi Queue dengan Linked List Program kali ini cukup istimewa karena menggunakan class sebagai dasar dari OOP(Object Oriented Programing) berikut source code dari program queue menggunakan linked list.
This is Queues Class
Struktur data queue (antrian) dapat diimplementasikan dengan menggunakan array maupun linked list sebagai penyimpanan datanya. Dalam contoh program berikut ini saya gunakan double linked list untuk implementasi queue.
1 Answer
Ok, so you have a couple of problems. I'm going to point out a few and let you work to fix the rest because this looks like an assignment and I don't want to do your homework for you :).
First, when you read from the file be careful not to ignore the first element:
Notice that unlike your solution I first do the push y.Push(line)
so that we don't forget to add whatever is already read into line
. Same goes for the queue file:
Just add it if it's not null
and then read the next line. You were always missing on the first element from the file.
Another problem is the Queues class (which by the way is misspelled you should replace O
with Q
). This one is not working properly because you forgot to increment and decrement the size when you insert or remove.
Notice that at the end of insert I'm increasing the size
so that the list
method doesn't throw a NullPointerException
every time we call it. Same goes for the remove
method:
Please also notice that your check before (if(head.nexttail)
) was also throwing NullPointerException
because at the beginning the head
is always null
so you cannot access the next
member. Finally I've made a small improvement to the list
method as well so that we return earlier:
Notice the return
if the Queue is empty, otherwise we will attempt to do tail.getNext()
which will always throw a NullPointerException
.
Some important thoughts about the code in generalPlease avoid weird naming. Why Queues? There is just one so it should be Queue.Please avoid weird variable names. Your code is not just for you, chances are someone else might need to read and it gets hard to know who it is s, y, k, fwy and fwk
. Why not naming them like this:
And the same goes for methods . Why Push
, Pop
and Top
are the only methods that start with upper-case letter ? If you don't agree with the standard Java naming convention that is fine but at least be consistent :).
Try the suggested improvements and see how your program it's working. I'm almost sure there are more problems with it. If you can't figure them out yourself leave a comment and I will help you. Good luck!

Not the answer you're looking for? Browse other questions tagged javadata-structures or ask your own question.
A data structure, or abstract data type (ADT), is a model that is defined by a collection of operations that can be performed on itself and is limited by the constraints on the effects of those operations. It creates a wall between what can be done to the underlying data and how it is to be done.
Most of us are familiar with stacks and queues in normal everyday usage, but what do supermarket queues and vending machines have to do with data structures? Let’s find out. In this article I’ll introduce you to two basic abstract data types – stack and queue – which have their origins in everyday usage.
Stacks
In common usage, a stack is a pile of objects which are typically arranged in layers – for example, a stack of books on your desk, or a stack of trays in the school cafeteria. In computer science parlance, a stack is a sequential collection with a particular property, in that, the last object placed on the stack, will be the first object removed. This property is commonly referred to as last in first out, or LIFO. Candy, chip, and cigarette vending machines operate on the same principle; the last item loaded in the rack is dispensed first.
In abstract terms, a stack is a linear list of items in which all additions to (a “push”) and deletions from (a “pop”) the list are restricted to one end – defined as the “top” (of the stack). The basic operations which define a stack are:
- init – create the stack.
- push – add an item to the top of the stack.
- pop – remove the last item added to the top of the stack.
- top – look at the item on the top of the stack without removing it.
- isEmpty – return whether the stack contains no more items.
A stack can also be implemented to have a maximum capacity. If the stack is full and does not contain enough slots to accept new entities, it is said to be an overflow – hence the phrase “stack overflow”. Likewise, if a pop operation is attempted on an empty stack then a “stack underflow” occurs.
Knowing that our stack is defined by the LIFO property and a number of basic operations, notably push and pop, we can easily implement a stack using arrays since arrays already provide push and pop operations.
Here’s what our simple stack looks like:
In this example, I’ve used array_unshift()
and array_shift()
, rather than array_push()
and array_pop()
, so that the first element of the stack is always the top. You could use array_push()
and array_pop()
to maintain semantic consistency, in which case, the Nth element of the stack becomes the top. It makes no difference either way since the whole purpose of an abstract data type is to abstract the manipulation of the data from its actual implementation.
Let’s add some items to the stack:
To remove some items from the stack:
Let’s see what’s at the top of the stack:
What if we remove it?
And if we add a new item?
You can see the stack operates on a last in first out basis. Whatever is last added to the stack is the first to be removed. If you continue to pop items until the stack is empty, you’ll get a stack underflow runtime exception.
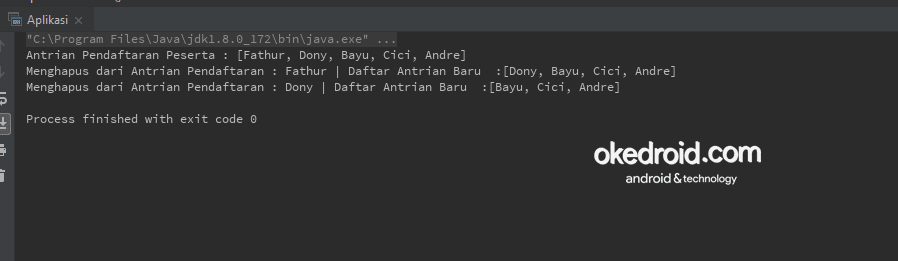
Contoh Program Queue Dengan Linked List
Oh, hello… PHP has kindly provided a stack trace showing the program execution call stack prior and up to the exception!
The SPLStack
The SPL extension provides a set of standard data structures, including the SplStack class (PHP5 >= 5.3.0). We can implement the same object, although much more tersely, using an SplStack as follows:
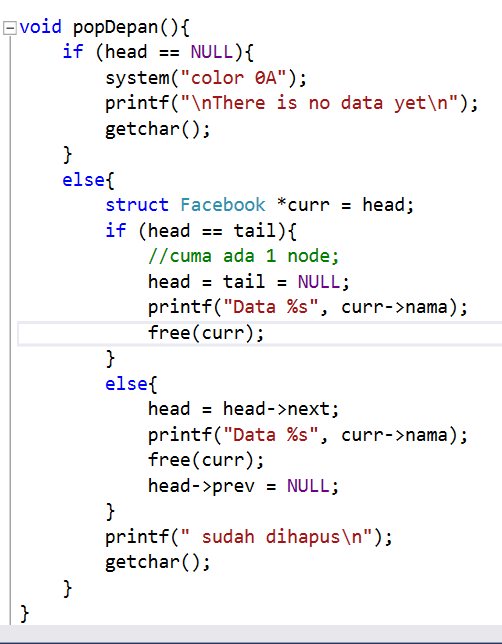
The SplStack
class implements a few more methods than we’ve originally defined. This is because SplStack
is implemented as a doubly-linked list, which provides the capacity to implement a traversable stack.
A linked list, which is another abstract data type itself, is a linear collection of objects (nodes) used to represent a particular sequence, where each node in the collection maintains a pointer to the next node in collection. In its simplest form, a linked list looks something like this:
Contoh Program Queue Dengan Linked List C++

In a doubly-linked list, each node has two pointers, each pointing to the next and previous nodes in the collection. This type of data structure allows for traversal in both directions.
Nodes marked with a cross (X) denotes a null or sentinel node – which designates the end of the traversal path (i.e. the path terminator).
Since ReadingList
is implemented as an SplStack
, we can traverse the stack forward (top-down) and backward (bottom-up). The default traversal mode for SplStack
is LIFO:
To traverse the stack in reverse order, we simply set the iterator mode to FIFO (first in, first out):
Queues
If you’ve ever been in a line at the supermarket checkout, then you’ll know that the first person in line gets served first. In computer terminology, a queue is another abstract data type, which operates on a first in first out basis, or FIFO. Inventory is also managed on a FIFO basis, particularly if such items are of a perishable nature.
The basic operations which define a queue are:
- init – create the queue.
- enqueue – add an item to the “end” (tail) of the queue.
- dequeue – remove an item from the “front” (head) of the queue.
- isEmpty – return whether the queue contains no more items.
Since SplQueue
is also implemented using a doubly-linked list, the semantic meaning of top and pop are reversed in this context. Let’s redefine our ReadingList
class as a queue:
SplDoublyLinkedList
also implements the ArrayAccess
interface so you can also add items to both SplQueue
and SplStack
as array elements:
To remove items from the front of the queue:
enqueue()
is an alias for push()
, but note that dequeue()
is not an alias for pop()
; pop()
has a different meaning and function in the context of a queue. If we had used pop()
here, it would remove the item from the end (tail) of the queue which violates the FIFO rule.
Similarly, to see what’s at the front (head) of the queue, we have to use bottom()
instead of top()
:
Summary
Contoh Program Queue Dengan Linked List Dan
In this article, you’ve seen how the stack and queue abstract data types are used in programming. These data structures are abstract, in that they are defined by the operations that can be performed on itself, thereby creating a wall between its implementation and the underlying data.
Contoh Program Queue Dengan Linked List Pdf
These structures are also constrained by the effect of such operations: You can only add or remove items from the top of the stack, and you can only remove items from the front of the queue, or add items to the rear of the queue.
Image by Alexandre Dulaunoy via Flickr